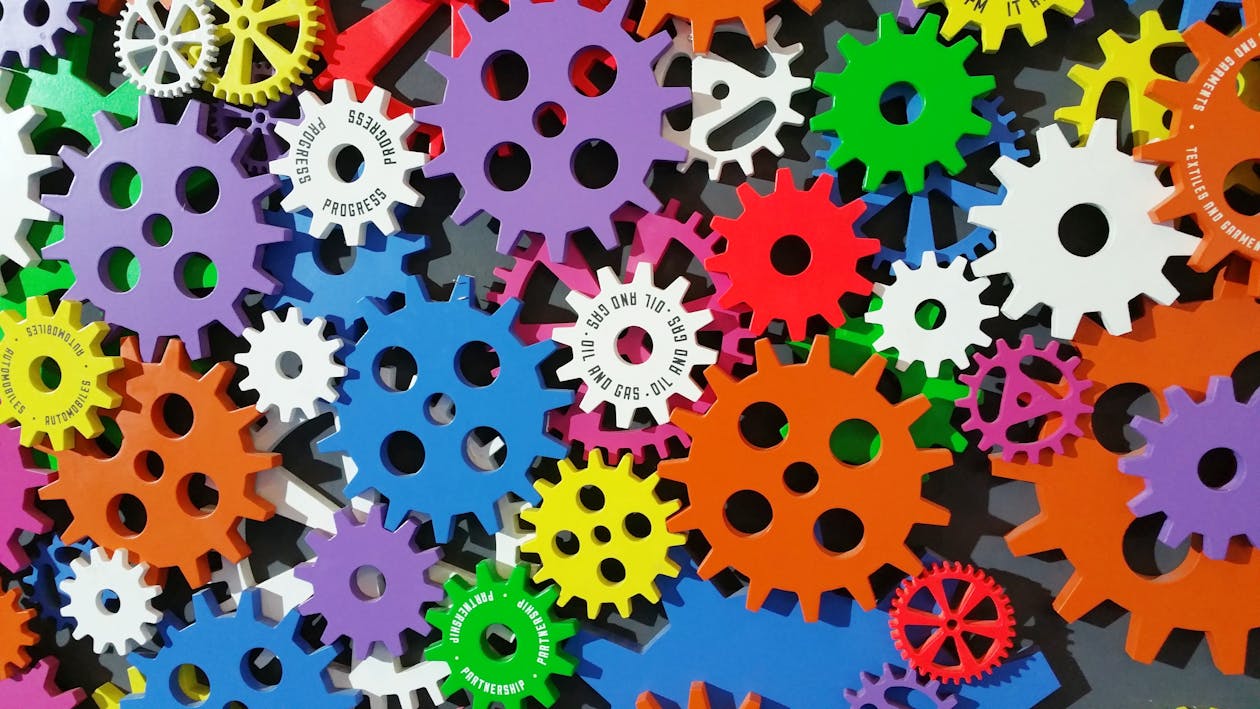
Automating Tasks with BASH, Python, and Cron Jobs on Linux Servers
In June 2024, I decided to automate my monthly tasks at work, which included running an Odoo script. This script, written in Python, interacts with Odoo's ORM (Object-Relational Mapping) system to search for invoices, approve them, and post them. Automating these tasks saves time, reduces errors, and improves efficiency. The Manual Process Previously, these scripts had to be run manually twice a month. This involved: 1. Login to the Server SSH into the production server. ssh <shortcut_name> Access the Odoo shell using a command with the -it flag to keep the session interactive and a require terminal. sudo docker exec -it <docker_name> /usr/bin/odoo shell --xmlrpc-port=<port> -d <database_name> --db_host <db_host> --db_password <db_password> --db_user <db_user> We use Amazon RDS for our database management and Docker for our application's environment. 2. Run the Script Manually start the script that processes the invoices.The script automates tasks such as approving and posting invoices. 3. Monitor the Script Ensure the computer running the script stays powered on and connected to the internet.Keep the shell session active to avoid interruptions.Continuously monitor the script to handle any unexpected issues. The process was prone to errors, particularly the could not obtain lock on row in realation "ir_sequence_date_range" error, which indicated database locking issues. This required manual retries, especially during peak hours. Need for Automation To streamline this process, I decided to automate it using BASH scripting, Python, and Cron Jobs on Linux servers. This approach would minimize manual intervention, reduces errors, and ensures consistent execution. Key Tools for Automation 1. Python Python is a versatile programming language known for its readability and extensive libraries. Python scripts handle more complex tasks, such as interacting with databases or web services. 2. BASH BASH (Bourne Again SHell) is a powerful scripting language for Unix-based systems. It can automate file operations, system monitoring, and more. 3. Cron Jobs Cron is a time-based job scheduler in Unix-like operating systems. It allows users to schedule scripts or commands to run automatically at specified times or intervals. Automating Odoo Tasks Here's how I automated the processing of invoices in Odoo: Step 1: Writing the Python Script First, I wrote a Python script to process the invoices in Odoo. The script searches for invoices, approves them, and posts them. Here's a simplified version of the script: # Search invoicesinvoices = env["account.move"].search([["type", "=", "out_invoice"]])for invoice in invoices: retries = 5 # Max retries while retries > 0: try: if len(invoice.invoice_line_ids) > 0: invoice.action_approve() invoice.action_post() env.cr.commit() # Commit after processing each invoice break # Break the retry loop if successful except Exception as e: env.cr.rollback() # Rollback the current invoice's operations retries -= 1 if retries > 0: print(f"Retrying... Attempts left: {retries}") time.sleep(5) # Wait for 5 seconds before retrying else: print("Max retries reached. Moving to the next invoice.") continue # Proceed to the next invoice Step 2: Creating a BASH Script Next, I created a BASH script to run the Python script. #!/bin/bashLOGFILE="/path/to/your/<log_name.log>"# Run the Odoo shell command and redirect output to the log filesudo docker exec -i <docker_name> /usr/bin/odoo shell --xmlrpc-port=<port> -d <database_name> --db_host <db_host> --db_password <db_password> --db_user <db_user> < /path/to/<python_script_name.py> >> $LOGFILE 2>&1 In the BASH script, we only use the -i flag because we don't need an interactive terminal session; the output is logged to a file, and the script doesn't need user interaction. This setup ensures the script runs as scheduled, even if we log out, the computer is off, or there's no internet connection. The automation stays reliable and doesn't need manual intervention. Step 3: Scheduling the Task with Cron Finally, I used Cron to schedule the BASH script to run at specified intervals. This ensures the Python script executes automatically without manual intervention. To edit the Cron schedule, I used the crontab -e command and added an entry like the following: 0 12 10 * * /path/to/<bash_script_name.sh> Understanding the Cron Schedule: 0: Minute (0th minute)12: Hour (12 PM, noon)10: Day of the month (10th day)*: Month (every month)*: Day of the week (every day of the week) This entry schedules the BASH script to run at 12:00 PM on the 10th of every month. Challenges and Solutions During manual execution of scripts, we encountered several challenges, including: 1. Manual Monitoring and Intervention The script required continuous monitoring to handle unexpected issues, such as database locking errors. By automating the script execution and adding retry mechanisms, we reduced the need for manual intervention. 2. Error Handling A common error encountered was could not obtain lock on row in relation "ir_sequence_date_range". This error indicated a database locking issue, requiring manual retries. We improved the script to include error handling and retry logic to address this issue automatically. 3. Session Maintenance The script execution required the shell session to remain active. We ensure that the script runs in a persistent environment using BASH and Cron, eliminating the need for manual session maintenance. Conclusion Automating tasks with BASH, Python, and Cron jobs on Linux servers can significantly improve efficiency and reduce manual effort. By leveraging these tools, we automated the processing of invoices in Odoo, handling errors and ensuring consistent execution. This approach not only saves time but also minimizes errors, allowing us to focus on more critical tasks. Automating is a powerful strategy that can be applied to various scenarios beyond invoice processing. Whether you're managing databases, monitoring systems, or performing routine maintenance, the combination of BASH, Python, and Cron can help streamline your workflows and enhance productivity.
Jul 26, 2024